Hi,
I am trying to detect deadlock in my console exe via ANTS performance profiler but I am unable to find any information on how to do that:
Here is the code for console exe:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
namespace DeadLockSimulator
{
class Program
{
private static object staticObjLockA = new Object();
private static object staticObjLockB = new Object();
public static void Main()
{
// Initialize thread with address of DoWork1
Thread thread1 = new Thread(DoWork1);
// Initilaize thread with address of DoWork2
Thread thread2 = new Thread(DoWork2);
// Start the Threads.
thread1.Start();
thread2.Start();
thread1.Join();
thread2.Join();
// This statement will never be executed.
Console.WriteLine("Done Processing...");
}
private static void DoWork1()
{
lock (staticObjLockA)
{
Console.WriteLine("Trying to acquire lock on staticObjLockB");
// Sleep to yield.
Thread.Sleep(1000);
lock (staticObjLockB)
{
// This block will never be executed.
Console.WriteLine("In DoWork1 Critical Section.");
// Access some shared resource here.
}
}
}
private static void DoWork2()
{
lock (staticObjLockB)
{
Console.WriteLine("Trying to acquire lock on staticObjLockA");
lock (staticObjLockA)
{
// This block will never be executed.
Console.WriteLine("In DoWork2 Critical Section.");
// Access some shared resource here.
}
}
}
}
}
Attached is the output for that exe:
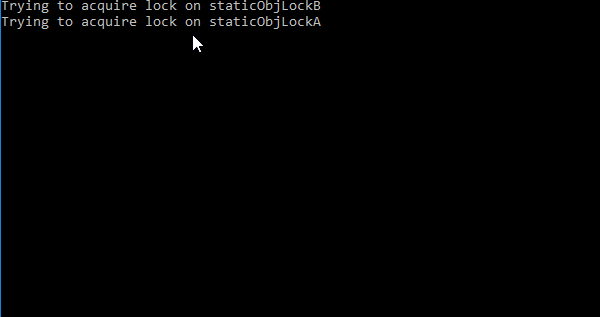
As you can notice the following piece of code is never executed because of a deadlock scenario:
Console.WriteLine("In DoWork1 Critical Section.");
Can you share some information on how to detect a deadlock scenario with your software?
I am trying to detect deadlock in my console exe via ANTS performance profiler but I am unable to find any information on how to do that:
Here is the code for console exe:
Attached is the output for that exe:
As you can notice the following piece of code is never executed because of a deadlock scenario:
Can you share some information on how to detect a deadlock scenario with your software?